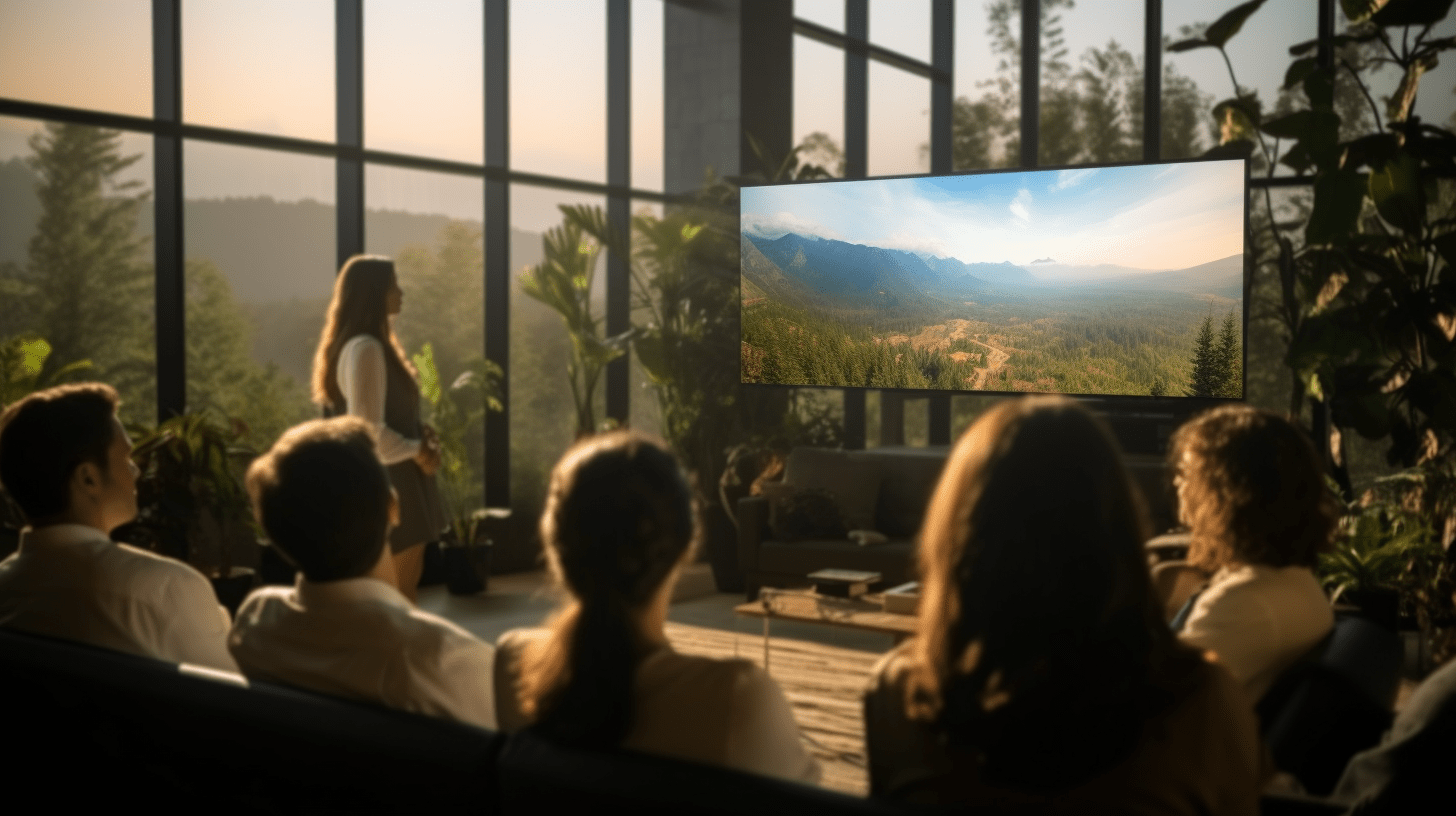
This article introduces the basics of Docker, explains how create an image Docker for a Streamlit application, and uses Docker Compose For create a WordPress site linked to a MySQL database. It also presents commands for creating, starting, stopping, and deleting containers.

Introduction
The objective of this tutorial is to introduce the basics of Docker. To do this, we will discover step by step the basic commands allowing us to put a Streamlit application into production. Then we will use Docker Compose to create a WordPress site by linking it to a MySQL database.
This tutorial is mainly aimed at programmers wishing to discover Docker but remains accessible to ordinary people.
Docker is a tool for launching and sharing an application easily, quickly and on any classic operating system (Windows, Linux, Mac and servers). To do this, it is based on the principle of image and container.
The objective of a container is the same as for a virtual machine: to host services (applications) on the same physical server while isolating them from each other. A container is, however, less fixed than a virtual machine in terms of disk size and allocated resources, but at the expense of slightly less isolation.
Even though each container is isolated, it is possible to make them communicate with each other using virtual networks. This makes it possible, for example, to link the container containing your application to the container containing your database.
If you want to install Docker, you can do so from the site Docker. If you cannot install Docker (administrator rights are required) or if you want to give it a quick try, the platform Play with Docker allows you to discover this tool online.
Create and launch an image
To create an image, you must first choose the image on which our application will be based. You can explore the images available on Docker Hub. In Data Science, we regularly use the Python image. This is the one we will use for the rest of this part.
We will create a docker image allowing you to launch an application Streamlit in Python. Our application will contain the following files:
-
- requirements.txt -> This must contain the necessary packages. Here, at least Streamlit.
- my_app.py -> Our Streamlit application. If you need an example application, you can deploy the Streamlit cheatsheet.
- Dockerfile -> Docker configuration file. We will create it.
Dockerfile
The Dockerfile is the file that Docker will use to obtain its instructions. In the case of our Streamlit application, the following elements will need to be specified in the Dockerfile:
-
- The base image to use. In our case we will use Python 3.10 alpine
- The port that the container will listen to when running. Here port 8501
- Workdir which allows you to modify the current directory. The command is equivalent to a command “ CD » on the command line. All subsequent commands will all be executed from the defined directory.
- We copy the requirements.txt present in our application into the container with the command “COPY”
- Once copied into the container, we execute the command “pip install – r requirements.txt” which allows you to install all the libraries present in the requirements.txt file. To do this we use the command “RUN”
- Once the libraries are installed, we copy the rest of the application into the container using “COPY”.
- Finally, we execute the command “streamlit run my_app.py” with "CMD”. This allows us to start our Streamlit application in the container.
This gives the following Dockerfile:
FROM python:3.10–alpine
EXPOSED 8501
WORKDIR /app
COPY requirements.txt ./requirements.txt
RUN pip3 install –r requirements.txt
COPY . .
CMD streamlit run my_app.py
It is important to understand the difference between RUN and CMD:
-
- RUN is a stage of image construction. The container state after a RUN command will be integrated into the container image. A Docker file can have many RUN steps that overlap one another to build the image.
- CMD is the command that the container runs by default when you launch the built image. A Dockerfile will only use the last defined CMD.
Create your image with DockerBuild
Once our Dockerfile is created, we need to build the image. To do this, simply use the following command:
“docker build -t streamlit_app . »
The “-t” option adds the streamlit_app tag to our image. Pay close attention to the “.” » at the end of the command. It allows you to specify that you want to use the current directory for our application
Start a container with Docker run
Now that our image has been built, we can start it. To do this we use the following command:
“docker run -dp 8501:8501 streamlit_app”
The -d option allows you to launch the container in detached mode (in background). The -p option allows you to specify the input and output port. We find the value that we indicated in the Dockerfile.
NB: If you want to directly launch an image that is published on Docker Hub, you do not need to create a Dockerfile and launch a Docker build command. For example, if the go language mascot makes you want to try this language, you can use the “docker run golang” command. This will start a command interface.
List, stop and delete images
List containers with docker ps
You can list active containers with the command “dockerps”.
In the example below we have a container docker/getting-started in progress and the output of this command should return something like this:
CONTAINER ID | PICTURE | ORDER | CREATED | STATUS | PORTS | NAMES |
969f65bcecb2 | docker/getting-started | “nginx -g '…” | 8 seconds ago | Up 7 seconds | 80/tcp… | stunned_davinci |
To list containers that have been stopped, you can add the -a argument.
NB: The name of a container is defined when it is created with the –name option. If this is not given, a random name is generated.
Stop a running container with dockerstop
To stop a container but without deleting it, you must use the command dockerstop. This takes the ID or name of the container as an argument. For example, to stop the container from the previous section, you can use one of these two commands:
-
- docker stop 969f65bcecb2
- docker stop stupefied_davinci
Delete a container with docker rm
Once a container is stopped, it is possible to delete it with the command docker rm. This works like dockerstop.
If you want to stop and remove a container with a single command, you can use the -f option. For example:
-
- docker rm -f 969f65bcecb2
- docker rm -f stupefied_davinci
Docker Compose
Compound is a tool for defining and launching multiple containers at once. The most wizard of readers might ask, “But tell me Jamy, the containers are supposed to be isolated from each other. So how can they communicate? ". Well my dear reader, it's thanks to the principle of Networking.
To put it simply, if Docker has been informed that two containers belong to the same network, then they can communicate. Otherwise, they can't. This keeps our containers completely insulated except when necessary. You will find a more detailed explanation on the Docker tutorial.
It is possible to define and launch several containers at once with the “classic” Docker commands but this is more laborious and takes more time. The tutorial getting started (part 5 to 7) created by Docker covers this topic.
A faster alternative, and one that we recommend, is to use Docker Compose, which is based on a file in the format YAML.
Using Compose consists of 3 steps:
-
- Creating the Dockerfile as seen earlier
- Creating the docker-compose.yaml file
- Launch containers with `docker compose up`
Creating a docker-compose.yaml file is simple but it depends on what you want to do. Generally speaking, a docker-compose file should contain the following:
-
- The version of docker-compose. In the example below we use version “3”
- The service list (or container list). In our case, we have the “db” service and the “wordpress” service. In each of the services, we will define all the elements that can be useful such as the image, the port, the environment variables for this container, the volumes that it will use, etc. Obviously this varies depending on the image you want to create.
- The volumes that must be used (the database). Here db_data refers to MySQL database.
After the theory, it's time for practice with an example that would allow you to deploy a WordPress site using a mySQL database. To achieve this, a possible docker-compose is as follows:
version: '3'
services:
db:
picture: mysql:5.7
volumes:
– db_data:/var/lib/mysql
restart: always
environment:
MYSQL_ROOT_PASSWORD: somewordpress
MYSQL_DATABASE: wordpress
MYSQL_USER: wordpress
MYSQL_PASSWORD: wordpress
wordpress:
depends_on:
– db
picture: wordpress:latest
ports:
– “ 8000:80“
restart: always
environment:
WORDPRESS_DB_HOST: db:3306
WORDPRESS_DB_USER: wordpress
WORDPRESS_DB_PASSWORD: wordpress
WORDPRESS_DB_NAME: wordpress
volumes:
db_data: {}
You will find more details regarding the lines of this file on the OpenClassrooms training.
It is important to note that docker compose is preinstalled on Windows, Mac and Play with Docker but this is not the case on Linux. If necessary, there is a installation manual.
Share your image on Docker Hub
Docker makes it easy to host your images on your hub in order to share them with other people. To do this, you must:
- Register/login to the site Docker Hub
- Press the “Create Repository” button
- Give your image a name and leave the visibility in “Public”. For the rest of the example, we will give the name “repo_sur_docker_hub”. In its free version, Docker allows you to have a private directory
- Press the “Create” button
- On the terminal containing the image to publish (here “my_image”), use the following commands:
-
- docker tag my_image USERNAME/repo_on_docker_hub
- docker push USERNAME/repo_on_docker_hub
-
If everything went well, your image is published to Docker Hub. You can recover it using the following command:
“docker run -dp 3000:3000 USERNAME/repo_on_docker_hub”
For further…
Once Docker is mastered, there are other tools such as Kubernetes or Ansible that can help you improve your solutions.
Indeed, if once deployed, your application is a great success, increasing the number of users can quickly pose a problem. In fact, you risk facing two situations:
-
- When updating your application, you don't want to have any service interruptions. Imagine if your mailbox was inaccessible during the update.
- If your application has an average of 1000 visits per hour but a peak of 10000 visitors at 8 p.m., this could pose a problem because the architecture would not be able to accommodate such demand.
The use of Kubernetes and or Ansible may be a solution to these concerns. In addition to offering you flexibility, proper use of these tools will allow you to reduce the cost of your architecture.